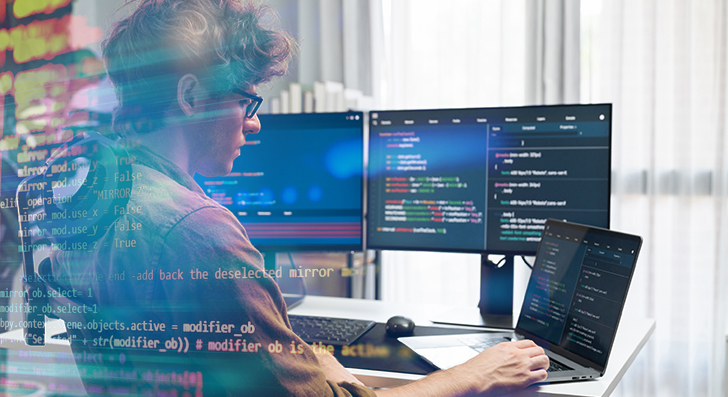
Scalability implies your application can deal with growth—extra people, far more info, and even more visitors—with out breaking. To be a developer, constructing with scalability in mind will save time and anxiety later. Right here’s a transparent and functional manual that will help you start by Gustavo Woltmann.
Style and design for Scalability from the Start
Scalability is just not something you bolt on later on—it should be part of your respective strategy from the start. Several purposes fail every time they expand quickly because the initial design can’t cope with the extra load. Being a developer, you have to Assume early about how your procedure will behave under pressure.
Start out by creating your architecture being flexible. Keep away from monolithic codebases where almost everything is tightly related. As an alternative, use modular style or microservices. These styles break your app into smaller, impartial sections. Every module or company can scale on its own without affecting The full method.
Also, contemplate your database from working day a single. Will it have to have to deal with 1,000,000 users or perhaps a hundred? Select the ideal type—relational or NoSQL—depending on how your knowledge will improve. Approach for sharding, indexing, and backups early, even if you don’t need to have them still.
A further vital point is to prevent hardcoding assumptions. Don’t compose code that only operates beneath recent ailments. Consider what would occur Should your user base doubled tomorrow. Would your app crash? Would the databases decelerate?
Use structure patterns that help scaling, like information queues or party-pushed systems. These assist your app deal with much more requests without having acquiring overloaded.
If you Establish with scalability in your mind, you are not just planning for achievement—you are lowering potential head aches. A effectively-planned procedure is less complicated to take care of, adapt, and increase. It’s greater to get ready early than to rebuild later on.
Use the correct Database
Choosing the right databases is usually a critical Section of creating scalable applications. Not all databases are designed precisely the same, and using the Incorrect you can sluggish you down or simply lead to failures as your app grows.
Begin by understanding your details. Can it be very structured, like rows in a desk? If Indeed, a relational databases like PostgreSQL or MySQL is an efficient suit. They are potent with associations, transactions, and regularity. Additionally they support scaling approaches like go through replicas, indexing, and partitioning to deal with more website traffic and info.
In the event your knowledge is a lot more versatile—like person activity logs, product or service catalogs, or documents—take into consideration a NoSQL selection like MongoDB, Cassandra, or DynamoDB. NoSQL databases are better at dealing with significant volumes of unstructured or semi-structured info and might scale horizontally more simply.
Also, think about your read through and write patterns. Are you undertaking many reads with fewer writes? Use caching and browse replicas. Will you be handling a large produce load? Look into databases that will take care of large produce throughput, or simply event-based mostly knowledge storage units like Apache Kafka (for temporary info streams).
It’s also sensible to Assume in advance. You might not need to have State-of-the-art scaling options now, but choosing a database that supports them indicates you gained’t need to have to change later on.
Use indexing to hurry up queries. Prevent pointless joins. Normalize or denormalize your information according to your accessibility designs. And constantly keep an eye on databases effectiveness when you mature.
To put it briefly, the right database is determined by your app’s structure, speed needs, and how you expect it to grow. Take time to select correctly—it’ll help save loads of hassle afterwards.
Enhance Code and Queries
Rapidly code is vital to scalability. As your app grows, every compact hold off adds up. Badly written code or unoptimized queries can decelerate performance and overload your system. That’s why it’s imperative that you Make successful logic from the start.
Start by crafting cleanse, basic code. Stay away from repeating logic and take away nearly anything avoidable. Don’t select the most sophisticated solution if a straightforward a single functions. Keep the features brief, concentrated, and simple to test. Use profiling instruments to uncover bottlenecks—spots exactly where your code usually takes way too lengthy to operate or makes use of too much memory.
Upcoming, take a look at your databases queries. These usually gradual points down over the code alone. Ensure Each individual query only asks for the info you actually will need. Stay away from Find *, which fetches every little thing, and in its place pick specific fields. Use indexes to speed up lookups. And stay clear of carrying out a lot of joins, especially across substantial tables.
In the event you detect the same knowledge remaining requested over and over, use caching. Shop the outcome quickly using equipment like Redis or Memcached therefore you don’t have to repeat pricey functions.
Also, batch your databases functions whenever you can. As an alternative to updating a row one after the other, update them in teams. This cuts down on overhead and helps make your application additional efficient.
Remember to check with massive datasets. Code and queries that get the job done fine with 100 records may well crash whenever they have to take care of one million.
In brief, scalable apps are rapidly applications. Maintain your code restricted, your queries lean, and use caching when wanted. These ways help your application stay smooth and responsive, even as the load increases.
Leverage Load Balancing and Caching
As your app grows, it has to manage far more people plus more targeted visitors. If everything goes through one server, it will quickly become a bottleneck. That’s where load balancing and caching are available. Both of these instruments help keep the application rapid, steady, and scalable.
Load balancing spreads incoming site visitors across numerous servers. In place of a person server executing the many operate, the load balancer routes consumers to various servers according to availability. This implies no single server receives overloaded. If one particular server goes down, the load balancer can ship traffic to the Many others. Instruments like Nginx, HAProxy, or cloud-based mostly options from AWS and Google Cloud make this straightforward to build.
Caching is about storing knowledge temporarily so it might be reused speedily. When customers request a similar facts once more—like an item website page or even a profile—you don’t need to fetch it with the database when. It is possible to serve it with the cache.
There are two popular varieties of caching:
1. Server-facet caching (like Redis or Memcached) retailers details in memory for rapidly access.
2. Shopper-aspect caching (like browser caching or CDN caching) suppliers static information near the consumer.
Caching cuts down database load, increases speed, and will make your app extra effective.
Use caching for things which don’t alter typically. And always be sure your cache is updated when facts does change.
In a nutshell, load balancing and caching are simple but effective applications. With each other, they assist your app take care of extra customers, keep speedy, and recover from troubles. If you propose to develop, you may need both of those.
Use Cloud and Container Tools
To create scalable apps, you need resources that allow your app improve easily. That’s in which cloud platforms and containers can be found in. They offer you adaptability, reduce setup time, and make scaling Significantly smoother.
Cloud platforms like Amazon World wide web Products and services (AWS), Google Cloud System (GCP), and Microsoft Azure let you rent servers and solutions as you will need them. You don’t really need to obtain hardware or guess long term capacity. When site visitors will increase, it is possible to incorporate far more methods with just a couple clicks or quickly applying vehicle-scaling. When traffic drops, you are able to scale down to save money.
These platforms also provide solutions like managed databases, storage, load balancing, and safety instruments. You may center on constructing your app as opposed to handling infrastructure.
Containers are An additional important tool. A container packages your application and anything it should run—code, libraries, settings—into a person device. This causes it to be simple to move your application involving environments, from the laptop to the cloud, without the need of surprises. Docker is the most well-liked Instrument for this.
When your application makes use of numerous containers, applications like Kubernetes make it easier to control them. Kubernetes handles deployment, scaling, and recovery. If just one portion of your application crashes, it restarts it quickly.
Containers also ensure it is easy to different areas of your application into companies. You are able to update or scale pieces independently, that's great for effectiveness and reliability.
Briefly, making use of cloud and container applications implies you can scale rapidly, deploy easily, and Get well quickly when problems come about. If you want your app to mature without having restrictions, begin working with these resources early. They help save time, reduce threat, and assist you remain centered on setting up, not fixing.
Keep an eye on All the things
Should you don’t watch your application, you gained’t know when matters here go Incorrect. Checking can help the thing is how your app is executing, place challenges early, and make much better choices as your application grows. It’s a vital A part of constructing scalable units.
Begin by tracking standard metrics like CPU utilization, memory, disk Place, and reaction time. These show you how your servers and solutions are executing. Applications like Prometheus, Grafana, Datadog, or New Relic will help you gather and visualize this info.
Don’t just keep an eye on your servers—watch your application much too. Regulate how much time it takes for users to load pages, how often errors occur, and in which they take place. Logging resources like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can assist you see what’s taking place inside your code.
Create alerts for crucial challenges. One example is, If the reaction time goes previously mentioned a limit or even a services goes down, you need to get notified instantly. This helps you fix challenges speedy, generally in advance of end users even recognize.
Monitoring is usually practical any time you make alterations. Should you deploy a brand new aspect and find out a spike in glitches or slowdowns, it is possible to roll it back before it will cause true harm.
As your application grows, website traffic and info increase. Devoid of monitoring, you’ll miss indications of difficulty right until it’s way too late. But with the proper applications in position, you stay on top of things.
In short, checking assists you keep the app responsible and scalable. It’s not nearly spotting failures—it’s about knowledge your program and ensuring that it works perfectly, even under pressure.
Remaining Ideas
Scalability isn’t only for large corporations. Even little applications want a solid foundation. By coming up with cautiously, optimizing correctly, and using the proper applications, you'll be able to Establish apps that increase effortlessly without having breaking stressed. Start tiny, Believe massive, and build wise.